Tutorial on finger-ready recognition
In this tutorial, we shall explain the basics of classifying an image into two categories of "finger ready for taking fingerprinting" and "finger not ready".
Contents
Preprocessing
Before we start, let's add necessary toolboxes to the search path of MATLAB:
addpath d:/users/jang/matlab/toolbox/utility addpath d:/users/jang/matlab/toolbox/machineLearning scriptStartTime=tic;
Dataset construction
First of all, the dataset is stored in a folder and available via this link. We can collect all the images using "mmDataCollect". The function will also display images of different classes in different figure windows, as follows:
imDir='fingerImage'; opt=mmDataCollect('defaultOpt'); opt.extName='jpg'; imData=mmDataCollect(imDir, opt, 1);
Collecting 306 files with extension "jpg" from "fingerImage"... Warning: Image is too big to fit on screen; displaying at 6% Warning: Image is too big to fit on screen; displaying at 6%
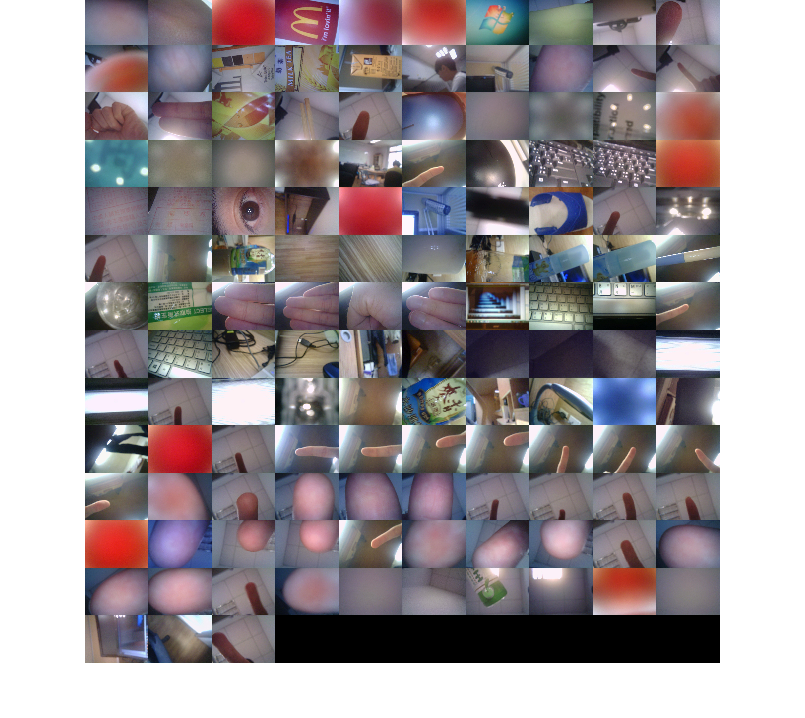
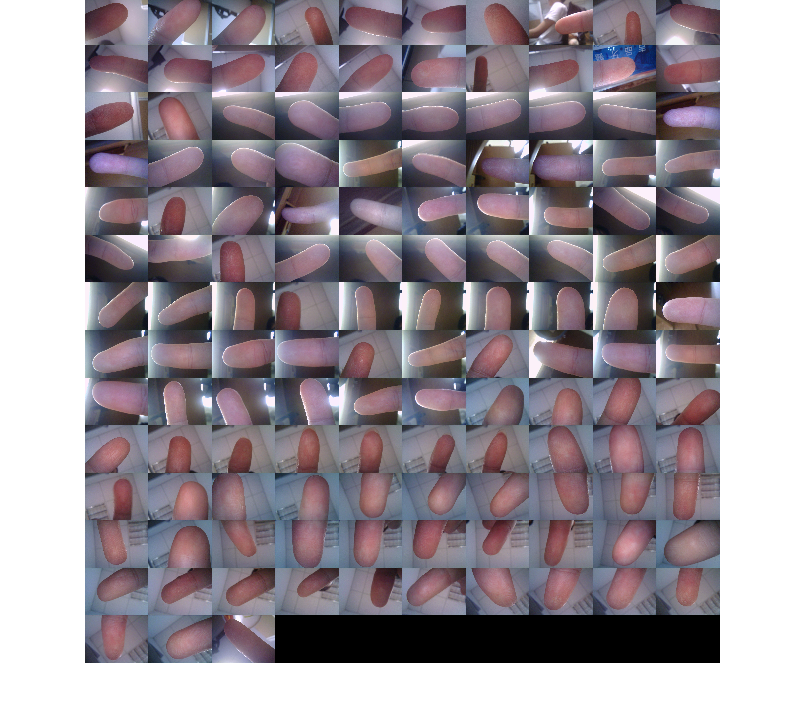
We need to perform feature extraction and put all the dataset into a format that is easier for further processing, including classifier construction and evaluation.
opt=dsCreateFromMm('defaultOpt'); if exist('ds.mat', 'file') fprintf('Loading ds.mat...\n'); load ds.mat else opt.imFeaFcn=@imFeaSkinRegionProp; % Function for feature extraction opt.imFeaOpt=feval(opt.imFeaFcn, 'defaultOpt'); % Feature options ds=dsCreateFromMm(imData, opt); fprintf('Saving ds.mat...\n'); save ds ds end
Loading ds.mat...
Note that in the above code snippet, if ds.mat exists, then we load it directly. On the other hand, if ds.mat does not exist, then we need to perform feature extraction and create the structure variable "ds" (and save it as ds.mat) for further processing.
Let's display the range of the inputs (features):
figure; dsRangePlot(ds);
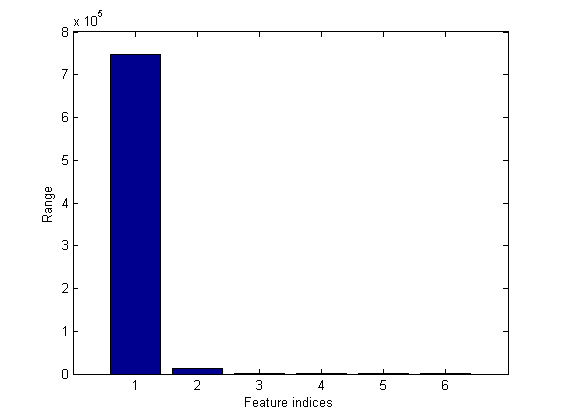
To further examine the distributions of the inputs using the box plot:
figure; dsBoxPlot(ds);
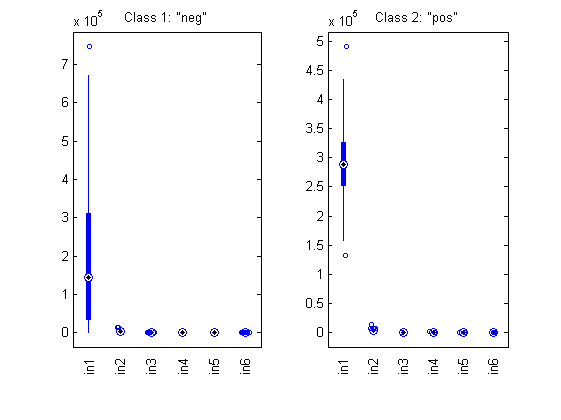
Apparently the range of the inputs vary a lot. We can perform z normalization to make the distribution easy to see:
ds2=ds; ds2.input=inputNormalize(ds2.input); figure; dsBoxPlot(ds2);
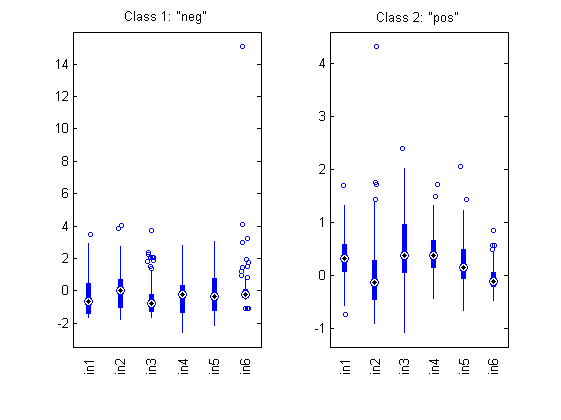
For tutorial purpose, we shall still use the original dataset (without normalization) for further processing.
Dimensionality reduction via PCA
The dimension of the feature vector is quite large:
dim=size(ds.input, 1);
We shall consider dimensionality reduction via PCA (principal component analysis). First, let's plot the cumulative variance given the descending eigenvalues of PCA:
[ds.input, eigVec, eigValue]=pca(ds.input); cumVar=cumsum(eigValue); cumVarPercent=cumVar/cumVar(end)*100; figure; plot(cumVarPercent, '.-'); xlabel('No. of eigenvalues'); ylabel('Cumulated variance percentage (%)'); title('Variance percentage vs. no. of eigenvalues');
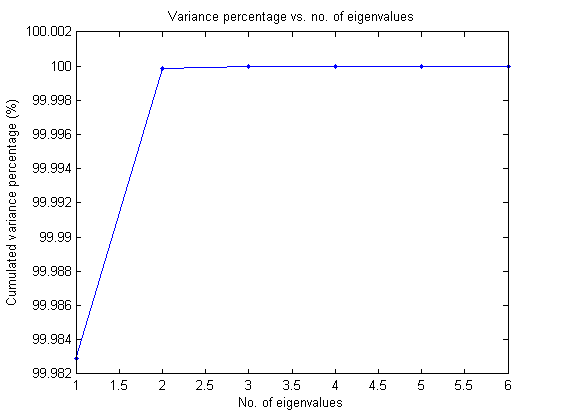
A reasonable choice is to retain the dimensionality such that the cumulative variance percent is larger than a given threshold:
index=find(cumVarPercent>opt.cumVarTh); newDim=index(1); %ds.input=ds.input(1:newDim, :); fprintf('Reduce the dimensionality to %d to keep %g%% cumulative variance via PCA.\n', newDim, opt.cumVarTh);
Reduce the dimensionality to 1 to keep 90% cumulative variance via PCA.
However, in this dataset, since the dimensionality is not too high, we do not perform PCA. Instead, we can perform LDA directly to see how the LOO of KNNC varies with the dimensionality:
opt=ldaPerfViaKnncLoo('defaultOpt'); opt.mode='exact'; recogRate1=ldaPerfViaKnncLoo(ds, opt); ds2=ds; ds2.input=inputNormalize(ds2.input); % input normalization recogRate2=ldaPerfViaKnncLoo(ds2, opt); [featureNum, dataNum] = size(ds.input); plot(1:featureNum, 100*recogRate1, 'o-', 1:featureNum, 100*recogRate2, '^-'); grid on legend('Raw data', 'Normalized data', 'location', 'southeast'); xlabel('No. of projected features based on LDA'); ylabel('LOO recognition rates using KNNC (%)');
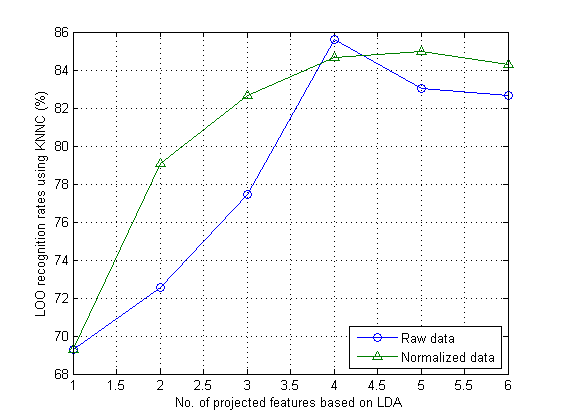
We can also perform input selection to reduce dimensionality. Here we are using leave-one-out cross validation based on SVM. Be aware that this is a time-consuming process:
inputSelectTic=tic;
z=inputSelectExhaustive(ds2, inf, 'svmc');
toc(inputSelectTic)
Construct 63 KNN models, each with up to 6 inputs selected from 6 candidates... modelIndex 1/63: selected={1} => Recog. rate = 82.679739% modelIndex 2/63: selected={2} => Recog. rate = 66.339869% modelIndex 3/63: selected={3} => Recog. rate = 74.183007% modelIndex 4/63: selected={4} => Recog. rate = 60.784314% modelIndex 5/63: selected={5} => Recog. rate = 58.496732% modelIndex 6/63: selected={6} => Recog. rate = 62.091503% modelIndex 7/63: selected={1, 2} => Recog. rate = 88.888889% modelIndex 8/63: selected={1, 3} => Recog. rate = 87.908497% modelIndex 9/63: selected={1, 4} => Recog. rate = 83.660131% modelIndex 10/63: selected={1, 5} => Recog. rate = 84.640523% modelIndex 11/63: selected={1, 6} => Recog. rate = 83.986928% modelIndex 12/63: selected={2, 3} => Recog. rate = 79.084967% modelIndex 13/63: selected={2, 4} => Recog. rate = 72.222222% modelIndex 14/63: selected={2, 5} => Recog. rate = 76.143791% modelIndex 15/63: selected={2, 6} => Recog. rate = 70.915033% modelIndex 16/63: selected={3, 4} => Recog. rate = 73.856209% modelIndex 17/63: selected={3, 5} => Recog. rate = 71.895425% modelIndex 18/63: selected={3, 6} => Recog. rate = 73.529412% modelIndex 19/63: selected={4, 5} => Recog. rate = 68.627451% modelIndex 20/63: selected={4, 6} => Recog. rate = 69.934641% modelIndex 21/63: selected={5, 6} => Recog. rate = 62.745098% modelIndex 22/63: selected={1, 2, 3} => Recog. rate = 90.522876% modelIndex 23/63: selected={1, 2, 4} => Recog. rate = 88.235294% modelIndex 24/63: selected={1, 2, 5} => Recog. rate = 88.888889% modelIndex 25/63: selected={1, 2, 6} => Recog. rate = 88.562092% modelIndex 26/63: selected={1, 3, 4} => Recog. rate = 87.254902% modelIndex 27/63: selected={1, 3, 5} => Recog. rate = 89.869281% modelIndex 28/63: selected={1, 3, 6} => Recog. rate = 87.581699% modelIndex 29/63: selected={1, 4, 5} => Recog. rate = 83.986928% modelIndex 30/63: selected={1, 4, 6} => Recog. rate = 84.967320% modelIndex 31/63: selected={1, 5, 6} => Recog. rate = 86.274510% modelIndex 32/63: selected={2, 3, 4} => Recog. rate = 81.372549% modelIndex 33/63: selected={2, 3, 5} => Recog. rate = 81.045752% modelIndex 34/63: selected={2, 3, 6} => Recog. rate = 81.372549% modelIndex 35/63: selected={2, 4, 5} => Recog. rate = 81.699346% modelIndex 36/63: selected={2, 4, 6} => Recog. rate = 75.490196% modelIndex 37/63: selected={2, 5, 6} => Recog. rate = 76.470588% modelIndex 38/63: selected={3, 4, 5} => Recog. rate = 76.470588% modelIndex 39/63: selected={3, 4, 6} => Recog. rate = 75.163399% modelIndex 40/63: selected={3, 5, 6} => Recog. rate = 75.163399% modelIndex 41/63: selected={4, 5, 6} => Recog. rate = 70.588235% modelIndex 42/63: selected={1, 2, 3, 4} => Recog. rate = 89.215686% modelIndex 43/63: selected={1, 2, 3, 5} => Recog. rate = 89.869281% modelIndex 44/63: selected={1, 2, 3, 6} => Recog. rate = 89.869281% modelIndex 45/63: selected={1, 2, 4, 5} => Recog. rate = 88.235294% modelIndex 46/63: selected={1, 2, 4, 6} => Recog. rate = 88.235294% modelIndex 47/63: selected={1, 2, 5, 6} => Recog. rate = 88.562092% modelIndex 48/63: selected={1, 3, 4, 5} => Recog. rate = 89.542484% modelIndex 49/63: selected={1, 3, 4, 6} => Recog. rate = 88.888889% modelIndex 50/63: selected={1, 3, 5, 6} => Recog. rate = 88.888889% modelIndex 51/63: selected={1, 4, 5, 6} => Recog. rate = 86.601307% modelIndex 52/63: selected={2, 3, 4, 5} => Recog. rate = 83.006536% modelIndex 53/63: selected={2, 3, 4, 6} => Recog. rate = 83.333333% modelIndex 54/63: selected={2, 3, 5, 6} => Recog. rate = 82.679739% modelIndex 55/63: selected={2, 4, 5, 6} => Recog. rate = 81.045752% modelIndex 56/63: selected={3, 4, 5, 6} => Recog. rate = 79.084967% modelIndex 57/63: selected={1, 2, 3, 4, 5} => Recog. rate = 89.215686% modelIndex 58/63: selected={1, 2, 3, 4, 6} => Recog. rate = 88.562092% modelIndex 59/63: selected={1, 2, 3, 5, 6} => Recog. rate = 89.542484% modelIndex 60/63: selected={1, 2, 4, 5, 6} => Recog. rate = 87.254902% modelIndex 61/63: selected={1, 3, 4, 5, 6} => Recog. rate = 90.196078% modelIndex 62/63: selected={2, 3, 4, 5, 6} => Recog. rate = 83.660131% modelIndex 63/63: selected={1, 2, 3, 4, 5, 6} => Recog. rate = 89.215686% Overall max recognition rate = 90.5%. Selected 3 inputs (out of 6): 1, 2, 3 Elapsed time is 2869.715333 seconds.
The accuracy of using inputs 1 and 2 are already pretty high, so we can have the scatter plots of all 2 inputs to visualize the separability:
ds3=ds2; ds3.input=ds3.input(1:2, :); figure; dsProjPlot2(ds3);
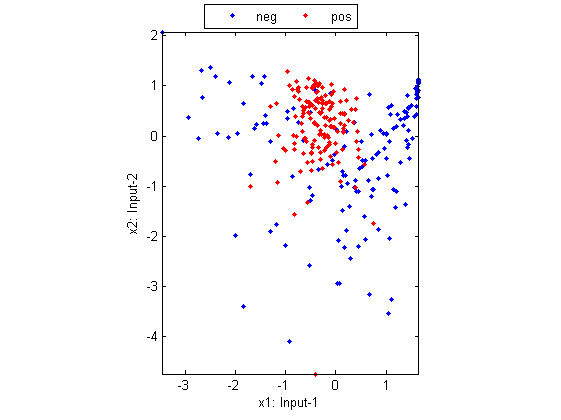
Performance evaluation
On the other hand, we can perform all combinations of classifiers and input normalization to search the best performance via leave-one-out cross validation:
poOpt=perfCv4classifier('defaultOpt'); poOpt.foldNum=inf; % Leave-one-out cross validation figure; [perfData, bestId]=perfCv4classifier(ds, poOpt, 1); structDispInHtml(perfData, 'Performance of various classifiers via cross validation');
Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=23557.7>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=97648.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=188072>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=170171>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=213913>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=210018>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=26207.9>10000 ===> Perhaps you should normalize the data first. Warning in gmmTrain: max(range)/min(range)=202749>10000 ===> Perhaps you should normalize the data first. Iteration=200/1000, recog. rate=46.8852% Iteration=400/1000, recog. rate=46.8852% Iteration=600/1000, recog. rate=46.5574% Iteration=800/1000, recog. rate=46.5574% Iteration=1000/1000, recog. rate=46.5574% Iteration=200/1000, recog. rate=68.1967% Iteration=400/1000, recog. rate=81.3115% Iteration=600/1000, recog. rate=82.2951% Iteration=800/1000, recog. rate=81.3115% Iteration=1000/1000, recog. rate=81.3115% Iteration=200/1000, recog. rate=41.6393% Iteration=400/1000, recog. rate=79.6721% Iteration=600/1000, recog. rate=78.3607% Iteration=800/1000, recog. rate=78.6885% Iteration=1000/1000, recog. rate=79.0164%
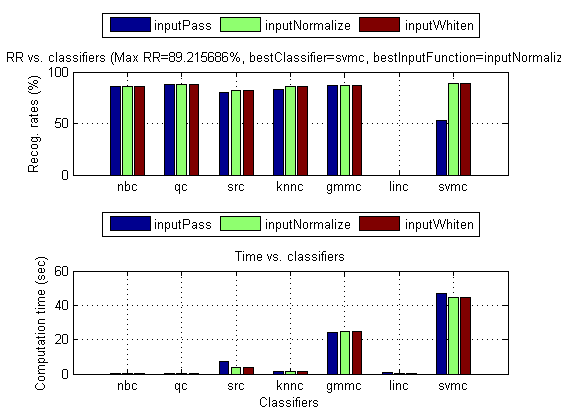
Confusion table and misclasification list
Then we can display the confusion matrix corresponding to the best classifier and the best input normalization scheme:
confMat=confMatGet(ds.output, perfData(bestId).bestComputedClass);
confOpt=confMatPlot('defaultOpt');
confOpt.className=ds.outputName;
figure; confMatPlot(confMat, confOpt);
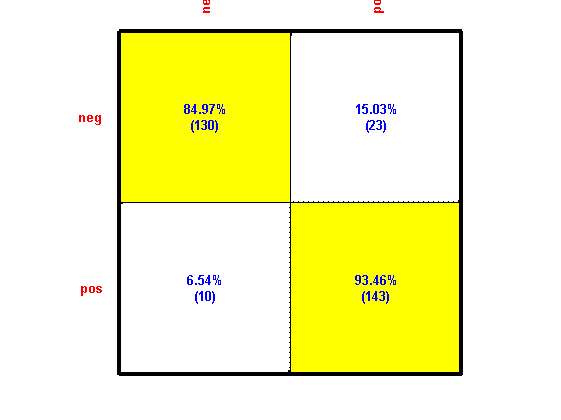
We can also list all the misclassified images in a table:
for i=1:length(imData) imData(i).classIdPredicted=perfData(bestId).bestComputedClass(i); imData(i).classPredicted=ds.outputName{imData(i).classIdPredicted}; end listOpt=mmDataList('defaultOpt'); mmDataList(imData, listOpt);
Summary
This is a brief tutorial on emotion recognition over image-based emoticons. There are several directions for further improvement:
- Explore other features
- Use other classifiers
Overall elapsed time:
toc(scriptStartTime)
Elapsed time is 3125.384035 seconds.
Jyh-Shing Roger Jang, created on
date
ans = 11-Jan-2015