Tutorial on emoticon recognition
In this tutorial, we shall explain the basics of emoticon recognition. More specifically, we shall construct a classifier that can classify a given emoticon into either positive or negative emotion.
Contents
Dataset preprocessing
Before we start, let's add necessary toolboxes to the search path of MATLAB:
addpath d:/users/jang/matlab/toolbox/utility addpath d:/users/jang/matlab/toolbox/machineLearning scriptStartTime=tic;
First of all, we need to collect emoticons that carry emotions. The collected emoticons can be display as follows:
emoticonDir='sourceEmoticon'; opt=fileList('defaultOpt'); opt.extName={'png', 'jpg'}; imData=fileList(emoticonDir, opt); for i=1:length(imData) im=imread(imData(i).path); figure; imshow(im); end
Warning: Image is too big to fit on screen; displaying at 67%
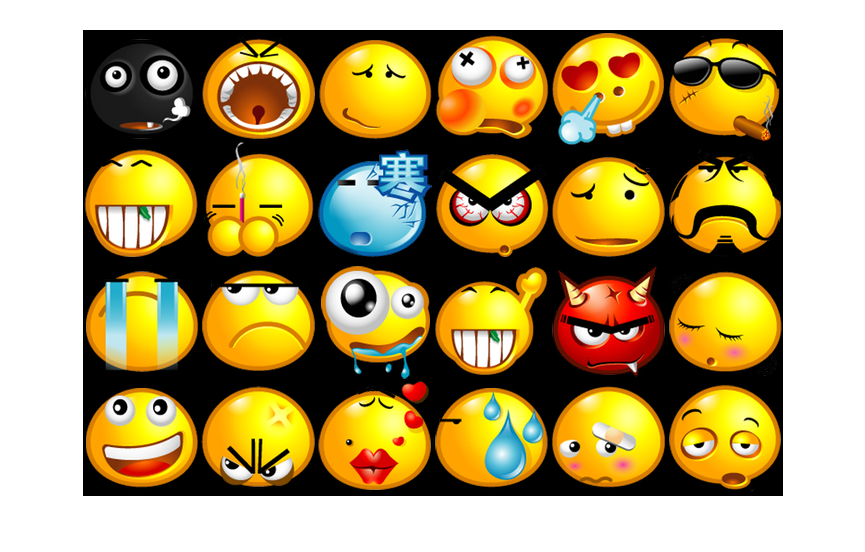
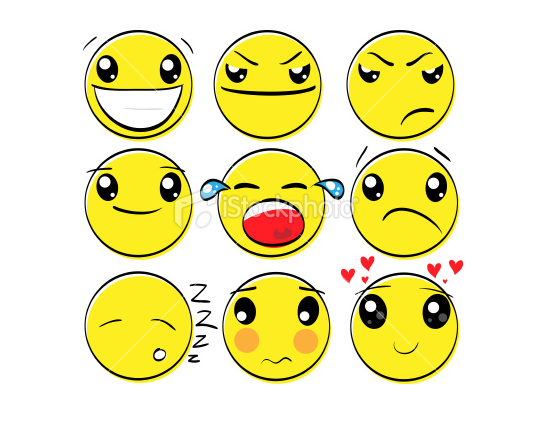
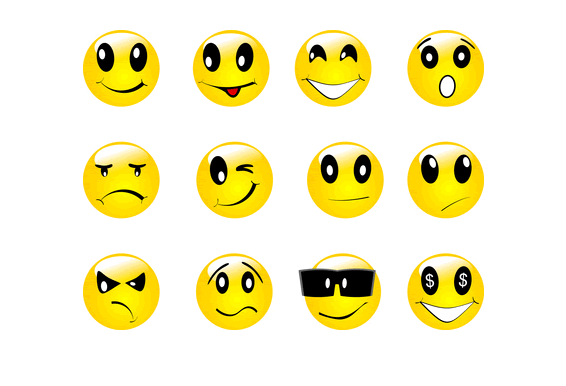
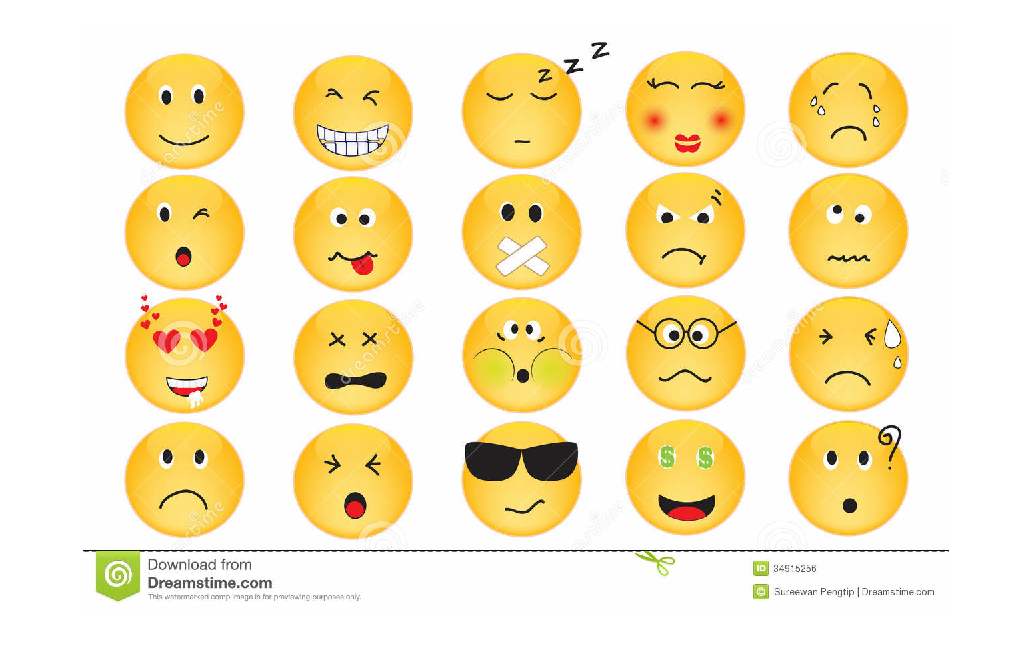
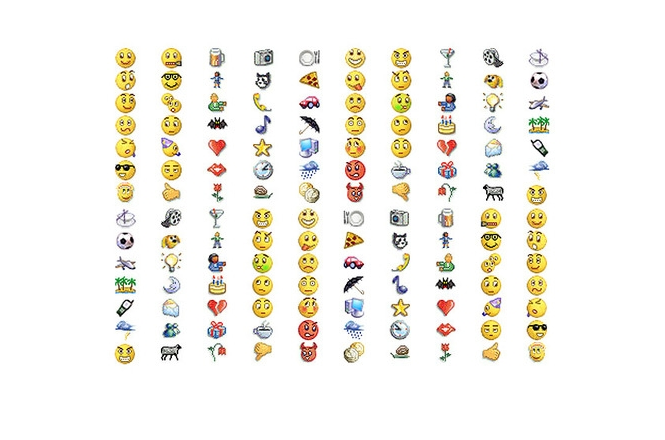
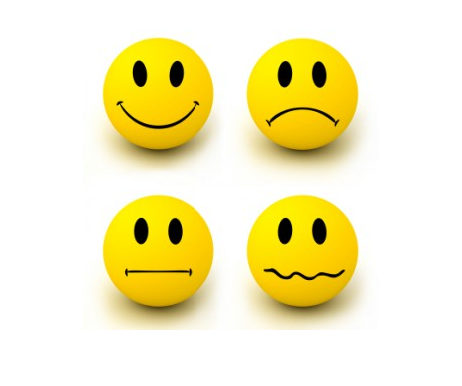
We can run the following program to crop each emoticon:
for i=1:length(imData); emoticonCrop(imData(i).path); end
Cropping images from sourceEmoticon/emotions.png... Cropping images from sourceEmoticon/set2.png... Cropping images from sourceEmoticon/set3.png... Cropping images from sourceEmoticon/set4.png... Cropping images from sourceEmoticon/emotions.jpg... Cropping images from sourceEmoticon/emotions2.jpg...
All the cropped images are stored in another folder "targetEmoticon. We can display the cropped image using the command "myMontage":
targetDir='targetEmoticon'; opt=fileList('defaultOpt'); opt.extName='png'; croppedImData=fileList(targetDir, opt); byte=[croppedImData.bytes]; bigImData=croppedImData(byte>1000); % Keep only big images [sortedValue, sortIndex]=sort([bigImData.bytes]); bigImData=bigImData(sortIndex); myMontage(bigImData);
Warning: Image is too big to fit on screen; displaying at 67%
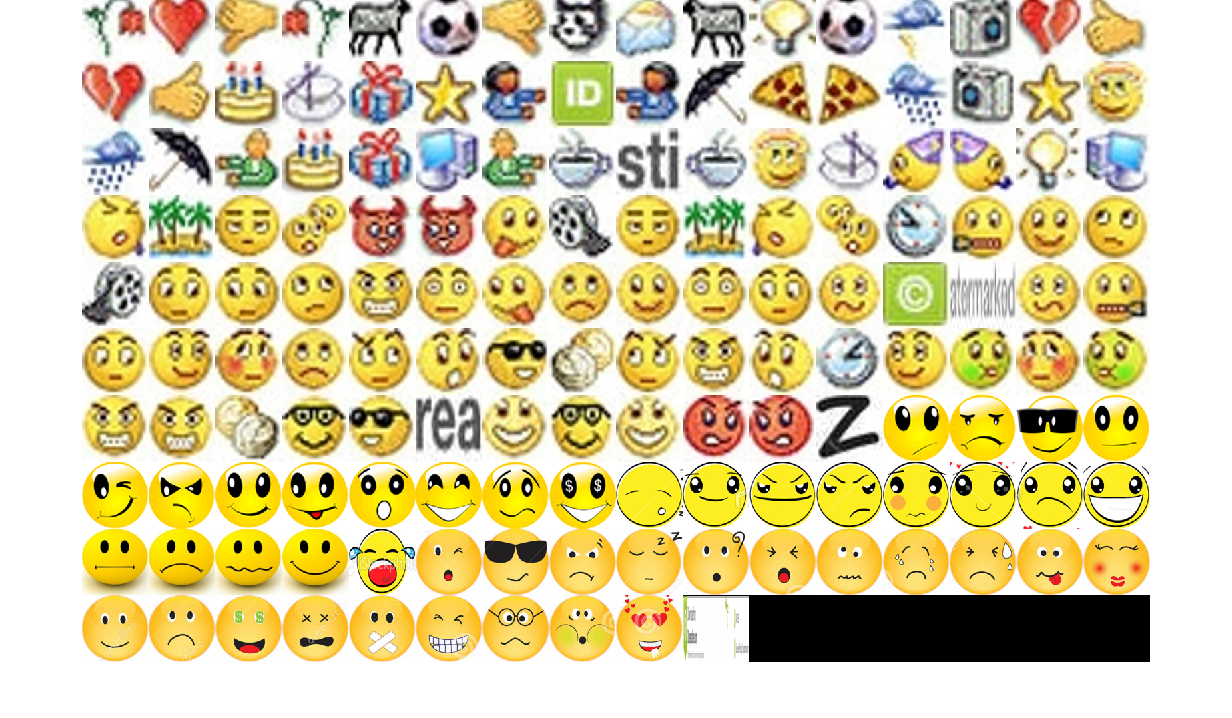
Since not all cropped image are valid emoticons, we need to manually select the ones for our classifier construction. More importantly, we need to label the groundtruth emotion of each emoticon. The manually collected dataset is available at this link. After this is done, we can collect all the images using "mmDataCollect". The function will also display images of different classes in different figure windows, as follows:
imDir='emoticon4training'; opt=mmDataCollect('defaultOpt'); opt.extName='png'; imData=mmDataCollect(imDir, opt, 1);
Collecting 102 files with extension "png" from "emoticon4training"...
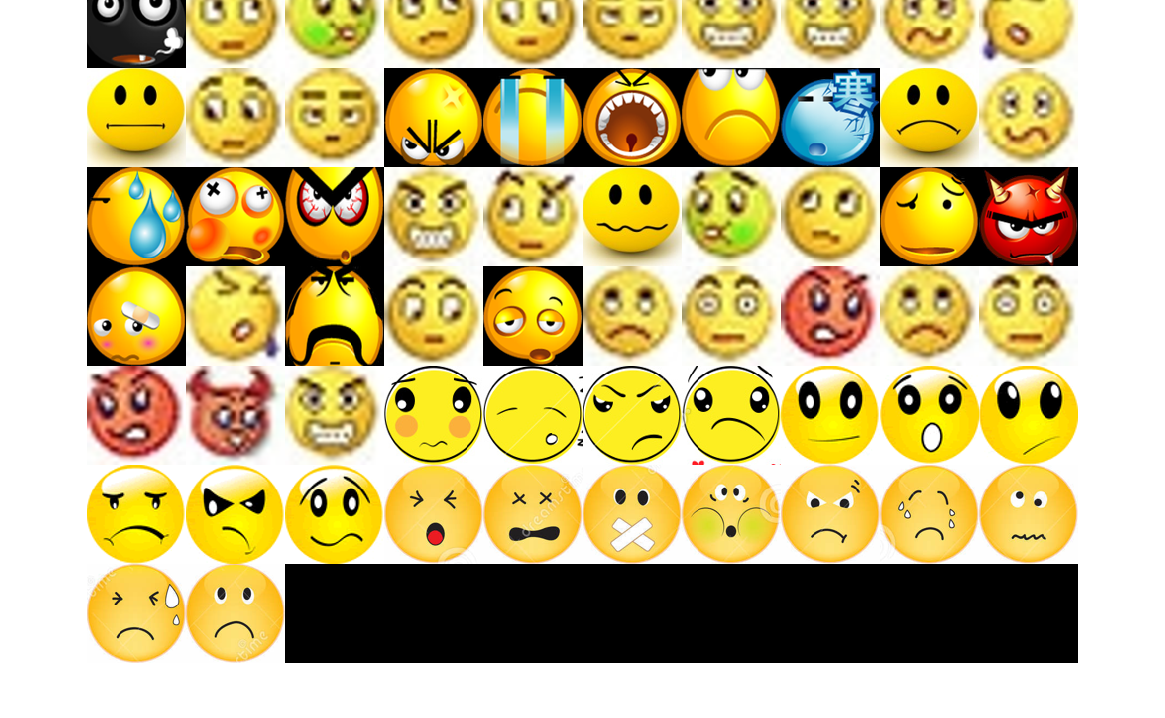
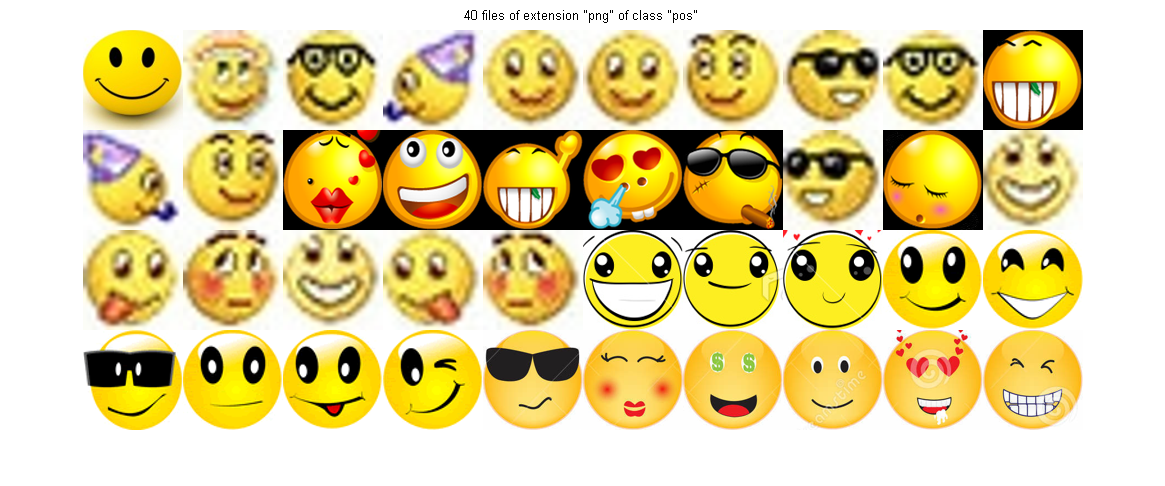
We need to perform feature extraction and put all the dataset into a format that is easier for further processing, including classifier construction and evaluation.
opt=dsCreateFromMm('defaultOpt'); opt.feaFcn=@imFeaLgbp; % Function for feature extraction if exist('ds.mat') fprintf('Loading ds.mat...\n'); load ds.mat else ds=dsCreateFromMm(imData, opt); fprintf('Saving ds.mat...\n'); save ds ds end
Loading ds.mat...
Note that in the above code snippet, if ds.mat exists, then we load it directly. On the other hand, if ds.mat does not exist, then we need to perform feature extraction and create the structure variable "ds" (and save it as ds.mat) for further processing.
Dimensionality reduction via PCA
The dimension of the feature vector is quite large:
dim=size(ds.input, 1)
dim = 655360
We shall consider dimensionality reduction via PCA (principal component analysis). First, let's plot the cumulative variance given the descending eigenvalues of PCA:
[ds.input, eigVec, eigValue]=pca(ds.input); cumVar=cumsum(eigValue); cumVarPercent=cumVar/cumVar(end)*100; figure; plot(cumVarPercent, '.-'); xlabel('No. of eigenvalues'); ylabel('Cumulated variance percentage (%)'); title('Variance percentage vs. no. of eigenvalues');
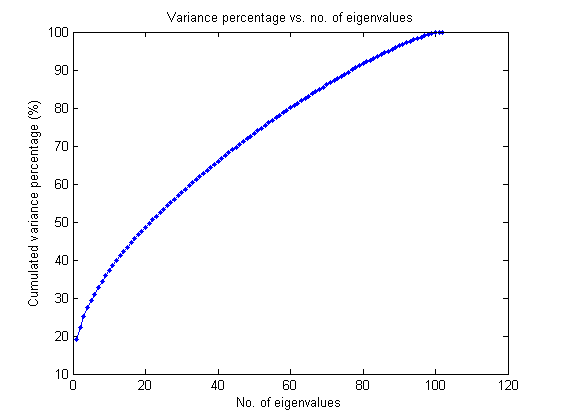
A reasonable choice is to retain the dimensionality such that the cumulative variance percent is larger than a given threshold:
opt=fcOptSet;
index=find(cumVarPercent>opt.cumVarTh);
newDim=index(1);
ds.input=ds.input(1:newDim, :);
fprintf('Reduce the dimensionality to %d to keep %g%% cumulative variance via PCA.\n', newDim, opt.cumVarTh);
Reduce the dimensionality to 77 to keep 90% cumulative variance via PCA.
Performance evaluation
After dimensionality reduction, we can perform all combinations of classifications and input normalization to search the best performance via leave-one-out cross validation:
poOpt=perfCv4classifier('defaultOpt'); poOpt.foldNum=inf; % Leave-one-out cross validation figure; [perfData, bestId]=perfCv4classifier(ds, poOpt, 1); structDispInHtml(perfData, 'Performance of various classifiers via cross validation');
Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.636317e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.377891e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.522694e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.133405e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.837185e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.387782e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.718908e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.557418e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.776026e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.000816e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.801659e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.060777e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.543224e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.066912e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.395800e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.322423e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.839535e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.491708e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.336052e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.479799e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.100730e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.228661e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.135922e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.437883e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.090075e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.498218e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.152020e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.277231e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.982044e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.005026e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.062353e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.358531e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.335831e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.592880e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.689588e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.470863e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.589033e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.057170e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.746721e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.665511e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.235853e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.512182e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.857516e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.644537e-22. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.398851e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.604272e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.245529e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.061769e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.331587e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.244148e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.920224e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.127070e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.501064e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.532877e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.383114e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.575676e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.854186e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.235546e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.555454e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.943975e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.976462e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.362191e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.486451e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.880239e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.360905e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.028747e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.880349e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.156782e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.742963e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.663461e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.043246e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.800591e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.547315e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.409478e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.887809e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.197524e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.837188e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.739398e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.890124e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.172172e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.211318e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.552909e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.478772e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.647280e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.720881e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.696377e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.357312e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.514694e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.796404e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.030723e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.568813e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.257227e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.610481e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.639377e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.316054e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.141503e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.319562e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.880148e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.177564e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.661433e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.306082e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.490869e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.485507e-20. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.884556e-20. Iteration=200/1000, recog. rate=59.4059% Iteration=400/1000, recog. rate=59.4059% Iteration=600/1000, recog. rate=58.4158% Iteration=800/1000, recog. rate=59.4059% Iteration=1000/1000, recog. rate=58.4158% Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.022943e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.203246e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.997706e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.684185e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.228517e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.582108e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.965636e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.289020e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.098602e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.038385e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.797820e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.633157e-18. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.409650e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.483658e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.527220e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.442557e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.212122e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.225131e-18. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.388042e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.641308e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.485278e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.577517e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.515768e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.094593e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.887347e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.117295e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.603632e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.063884e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.580100e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.953462e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.694440e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.433998e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.928557e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.276430e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.743378e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.973761e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.136168e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.252637e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.802372e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.537367e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.103826e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.134982e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.315746e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.168463e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.780940e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.110738e-18. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.245900e-18. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.444921e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.255898e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.928868e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.173792e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.783746e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.691180e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.662106e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.070767e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.492436e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.441696e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.262287e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.365512e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.191664e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.702452e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.143585e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.825495e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.427728e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.865934e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.626015e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.313452e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.320684e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.489428e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.757875e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.170031e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.148507e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.469528e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.987939e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.196051e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.541112e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.313385e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.740970e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.874860e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.746225e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.406088e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.931355e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.588684e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.187180e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.640722e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.147709e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.313733e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.746752e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.314014e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.518396e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.581933e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.530954e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.698804e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.367840e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.219684e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.568439e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.552716e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.054755e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.620507e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.041259e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.922627e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.271472e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.230667e-18. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.189645e-19. Iteration=200/1000, recog. rate=65.3465% Iteration=400/1000, recog. rate=72.2772% Iteration=600/1000, recog. rate=73.2673% Iteration=800/1000, recog. rate=72.2772% Iteration=1000/1000, recog. rate=73.2673% Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.136443e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.517749e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.005993e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.903798e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.355705e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.056658e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.683982e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.851674e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.873015e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.147154e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.282839e-18. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.597002e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.820791e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.593867e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.164531e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.454016e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.577812e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.070427e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.986174e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.745569e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.280652e-18. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.602325e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.541745e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.048629e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.702255e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.311384e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.592101e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.036712e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.681286e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.704500e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.044110e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.049795e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.586665e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.042213e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.503036e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.557634e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.870865e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.862180e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.504074e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.490110e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.422561e-18. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.771737e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.115862e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.268657e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.230368e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.066821e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.289445e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.998251e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.686279e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.000464e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.798674e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.141018e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.883350e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.635225e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.559889e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.488070e-20. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.562174e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.543297e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.161216e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.235912e-21. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.424649e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (61) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.548094e-19. Warning: Warning in gaussianMle: dataNum (40) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.864172e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.583528e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.267601e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.254382e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.714542e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.312635e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.453585e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.363761e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.389687e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 6.302428e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.189351e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.782013e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.660826e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.420604e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.759185e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 9.833120e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.895259e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.632678e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.920655e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.380053e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.020056e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 7.373313e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.792483e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.047637e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.810842e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.861088e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.538920e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 5.711439e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.437200e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.241340e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.210281e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.405168e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.808986e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.818767e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.348168e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.136997e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 4.798145e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 8.012113e-20. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 1.466996e-19. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 3.541788e-21. Warning: Warning in gaussianMle: dataNum (62) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.734913e-19. Warning: Warning in gaussianMle: dataNum (39) <= dim (77), the resulting parameters are not trustworthy! Warning: Matrix is close to singular or badly scaled. Results may be inaccurate. RCOND = 2.354502e-19. Iteration=200/1000, recog. rate=67.3267% Iteration=400/1000, recog. rate=74.2574% Iteration=600/1000, recog. rate=81.1881% Iteration=800/1000, recog. rate=85.1485% Iteration=1000/1000, recog. rate=85.1485%
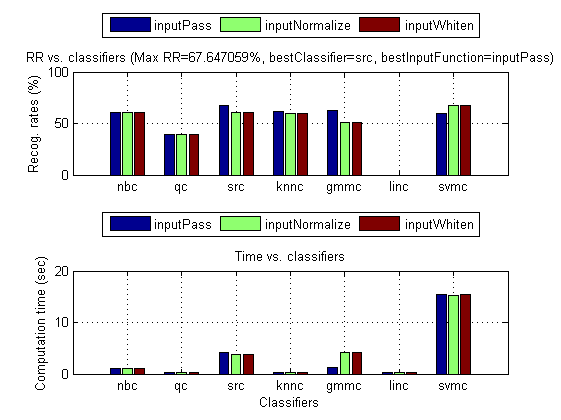
Confusion table and misclassification list
Then we can display the confusion matrix corresponding to the best classifier and the best input normalization scheme:
confMat=confMatGet(ds.output, perfData(bestId).bestComputedClass);
confOpt=confMatPlot('defaultOpt');
confOpt.className=ds.outputName;
figure; confMatPlot(confMat, confOpt);
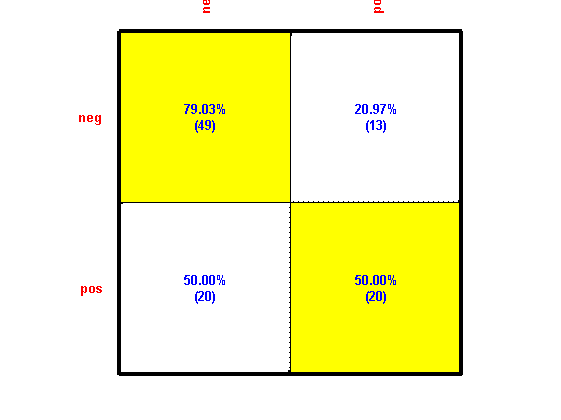
We can also list all the misclassified images in a table:
for i=1:length(imData) imData(i).classIdPredicted=perfData(bestId).bestComputedClass(i); imData(i).classPredicted=ds.outputName{imData(i).classIdPredicted}; end listOpt=mmDataList('defaultOpt'); mmDataList(imData, listOpt);
Summary
This is a brief tutorial on emotion recognition over image-based emoticons. There are several directions for further improvement:
- Try other method for automatic emoticon cropping and labeling
- Explore other features
- Use other classifiers
Overall elapsed time:
toc(scriptStartTime)
Elapsed time is 95.429224 seconds.
Jyh-Shing Roger Jang, created on
date
ans = 11-Jan-2015